The Law of Demeter is a very good software principle that can allow developers to write better testable code and limit the overall knowledge of the system between two stranger classes.
Each unit should have only limited knowledge about other units: only units “closely” related to the current unit.
Minimize Coupling
What’s wrong with having modules that know about each other? You do need to be careful about how many other modules you interact with and, more importantly, how you came to interact with them.
When we ask an object for a particular service, we’d like the service to be performed on our behalf. We do not want the object to give us a third-party object that we have to deal with to get the required service.
For example, suppose you are writing a class that generates a graph of scientific recorder data. You have data recorders spread around the world; each recorder object contains a location object giving its position and time zone. You want to let your users select a recorder and plot its data, labeled with the correct time zone. You might write
public void plotDate(Date aDate, Selection aSelection) {
TimeZone tz =
aSelection.getRecorder().getLocation().getTimeZone();
...
}
But now the plotting routine is unnecessarily coupled to three classes— Selection, Recorder, and Location. This style of coding dramatically increases the number of classes on which our class depends.
Why is this a bad thing? It increases the risk that an unrelated change somewhere else in the system will affect your code.
Systems with many unnecessary dependencies are very hard (and expensive) to maintain, and tend to be highly unstable. In order to keep the dependencies to a minimum, we’ll use the Law of Demeter to design our methods and functions.
The Law of Demeter for Functions
The Law of Demeter for functions attempts to minimize coupling between modules in any given program. It tries to prevent you from reaching into an object to gain access to a third object’s methods. The law can be summarized as:
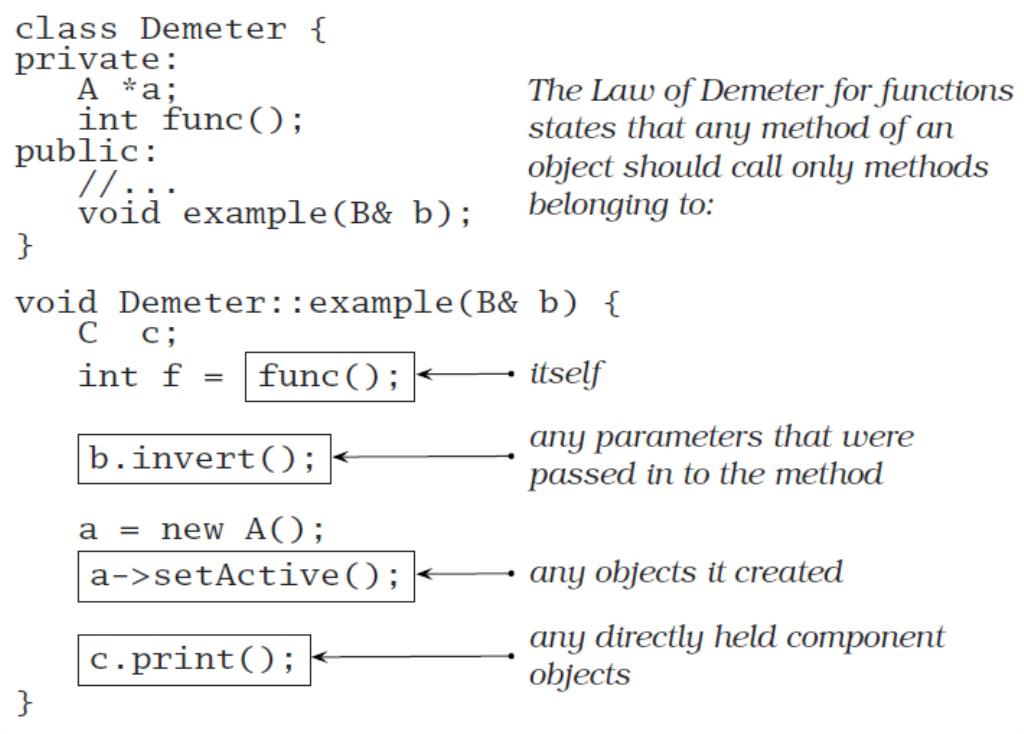
Using The Law of Demeter will make your code more adaptable and robust, but at a cost: as a “general contractor,” your module must delegate and manage any and all subcontractors directly, without involving
clients of your module. In practice, this means that you will be writing a large number of wrapper methods that simply forward the request on to a delegate. These wrapper methods will impose both a runtime cost
and a space overhead, which may be significant—even prohibitive—in some applications.
As with any technique, you must balance the pros and cons for your particular application.
Example of Violation
public void showBalance(BankAccount acct) {
Money amt = acct.getBalance();
printToScreen(amt.printFormat());
}
The variable acct is passed in as a parameter, so the getBalance call is allowed. Calling amt.printFormat(), however, is not. We don’t “own” amt and it wasn’t passed to us. We could eliminate showBalance’s coupling to Money with something like this:
void showBalance(BankAccount b) {
b.printBalance();
}
LoD and SOLID
What I like about the Law of Demeter is how nicely it wraps up many other practices of object oriented design. It enforces proper encapsulation by isolating changes in subobjects. This in turn helps to create better models of real world objects by putting pressure on the developer to improve interface design.
Single Responsibility Principle:
“have only one reason for change” - Breaking the Law of Demeter breaks the SRP because when a subobject changes, your object would have to change also.
Dependency Inversion:
“depend upon abstractions, not concretions” - so by not reaching into the guts of an object, you are forces to depend on an abstraction, not the nitty gritty details of the object.
IT
law of demeter LoD
14.09.2020
Acasa