First, we are going to start with Understanding the Different .NET Platforms, and then we'll flow into the unification of .NET happening with .NET 6. We had the .NET Framework introduced many years ago. This is still around and millions of applications have been built with it, and are still being built with it today. And currently, that is at version 4.8. That's the .NET that runs only on Windows.
Then, a few years ago, Microsoft acquired Xamarin. Xamarin is the framework that allows you to build mobile apps that run on iOS and android using C#, and it's based on Mono. Mono is the original open‑source version of .NET, which dates back to the early days of .NET 2. Although it's a different platform, the APIs are very similar, if not equal, meaning that you could write the same C# code, but now against Mono instead of the regular .NET.
And then there's .NET Core. .NET Core is introduced also as cross‑platform, but in a different way than Xamarin. Using .Net Core, .NET developers could write replications and websites to run on Windows, Linux, and Mac. .Net Core is where all the innovation happens. It is where Microsoft puts all its efforts into. So although we always talk about .NET, there are, in fact, three .NET platforms, and you can see them again here:
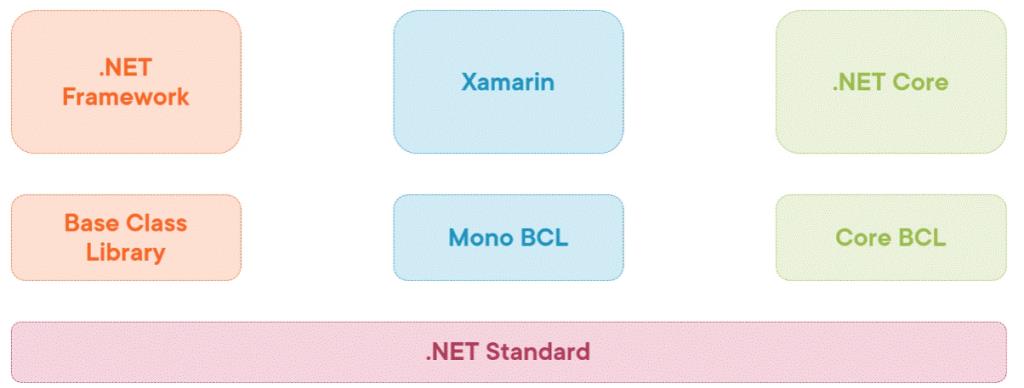
Each of these have their own BCL or base class library. The BCL is the library containing many thousands of classes which you use all the time when creating .NET applications. And although the libraries are very similar, they aren't identical, and thus, at least initially, we couldn't share code between the different silos. That, however, has been one of Microsoft's longstanding dreams, allowing developers to write code once and run it on multiple platforms. But because we had these different .NET platforms, that was not possible. Now, Microsoft has been working to give developers the ability to share code, and the most commonly used approach to allow us to share code between these different .NET platforms is .NET Standard. .NET Standard has been around for quite some time now, basically allowing us to create a library in which we write our code, and it can be referenced from different platforms. Microsoft guarantees that all that you write in there is understood by the different .NET platforms. Now, this is, of course, non‑UI code, as you can't share UI code between a mobile Xamarin application and a web application built in ASP.Net Core.
Microsoft is taking things a step further. Basically, with .NET 6, the different platforms are now being unified. We won't say .NET Core or .NET Framework anymore, all there is now is .NET 6, one .NET going forward. It is the same .NET that you'll be using to build all types of applications now, from desktop apps, to cloud apps, web apps, games with Unity, mobile apps, and AI. The amount of code that you can share is now pretty much at its maximum, allowing you to share pretty much everything. On top of this shared .NET, we will now have specific libraries you can bring in to add specific functionalities, such as, for example, when you're building a mobile app. But underneath, they're all running on the same .NET So with .NET 6, we're really seeing the different .NET platforms come together.
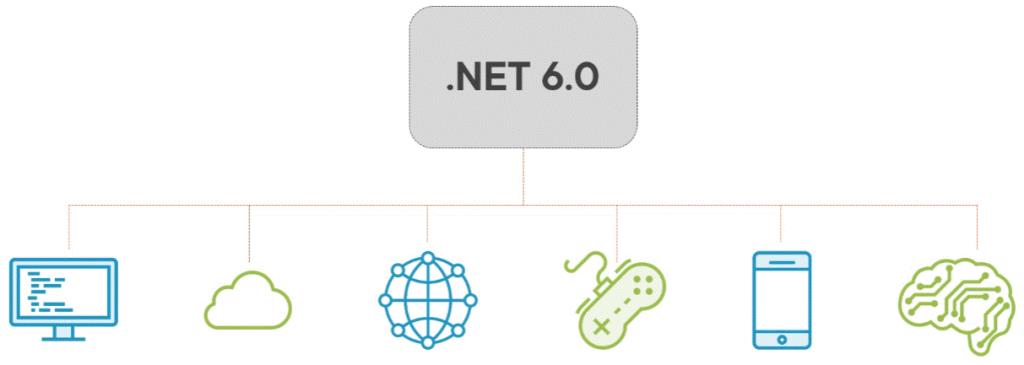
In fact, this unification process already started with .NET 5 and now completed basically with .NET 6. For us, as .NET developers, it means that we now have a single SDK and BCL. No different .NET platforms, no different BCLs, all is now on the same foundation.
C# 10
A new version of the C# language is being included as part of the .NET 6.0 release as well. We're now at C# 10, which comes with quite a lot of interesting changes. The BCL(Base Class Library) contains some interesting APIs that will help us making our code simpler and cleaner. The .NET 6 API changes and a new version of the C# language will be the topics of this module.
Global Using Statements
With C# 10, we're getting the ability to declare global namespaces, so globally across the entire project. When a namespace is imported globally, it's types are available everywhere. Yes, this can more easily now bring in name collisions; that is true. You can declare a using statement to be global in every class, so wherever you want, but I do recommend placing these global using statements in a separate file such as GlobalUsings.cs. For example, declares such a using anywhere in your C# files, and you don't need to add any individual using for the following using statement:

File Scoped Namespaces
The second important new C# feature is file‑scoped namespaces, or file‑based namespaces. Up to now we had namespaces just like in pretty much every code file you create, after your usings, you put the namespace. The namespace then is followed by a set of curly braces, which then results in the fact that all code between these will now be part of the namespace. So here our class, living between the curly braces, is now part of the namespace. And we do what we have been doing for years. All code is indented because it's inside of that namespace.
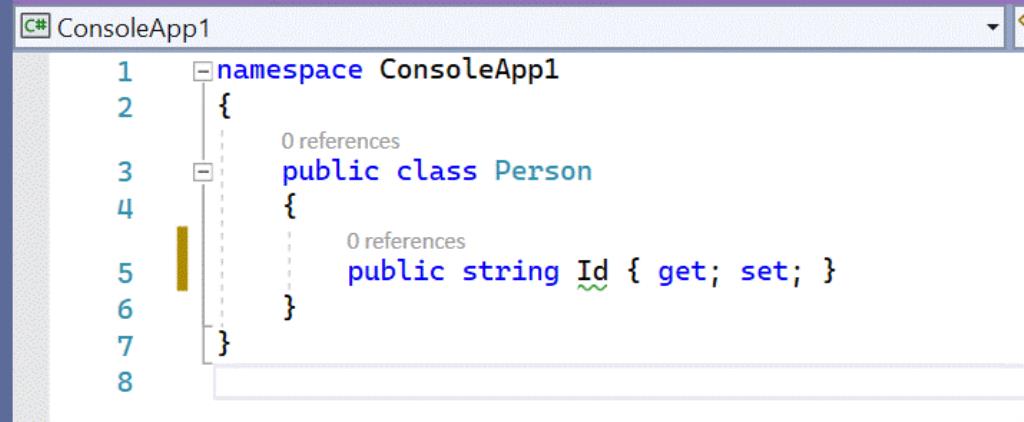
Now when we look at the possibility in .NET 6, we can see file‑scoped namespaces. Note the semicolon at the end. Now, and that's where the name comes from. All code in this file automatically becomes part of this namespace. What we are now gaining is horizontal spacing and perhaps also a bit less clutter.
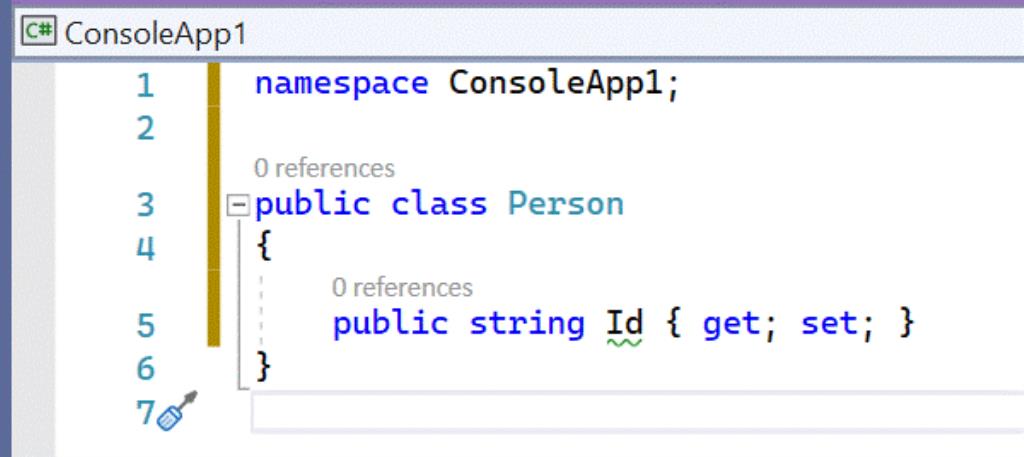
DateOnly and TimeOnly
.NET 6 comes with DateOnly and TimeOnly, two new types that do exactly what the name says they do. They allow us to create a struct that contains a date without the time part or the time without the date part. Let's see what this is all about in Visual Studio. .NET 6 comes with a few classes that we can use to represent a date only or the time only because so far we've always had to use the DateTime, which always contains both a date and a time part. Here is a simple Console application which demonstrates the DateOnly display value:
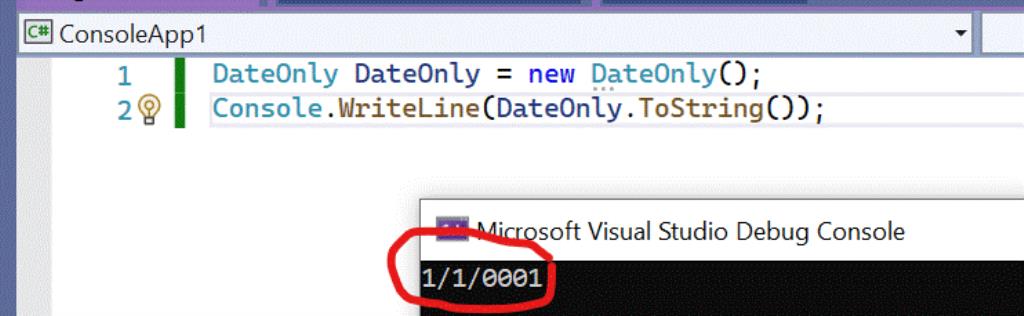
New LINQ Methods
There are new By operator methods, namely MinBy and MaxBy. MaxBy will return the maximum value in a generic sequence according to a specified key selector function. MinBy will do the same, but, of course, the minimum value. Here in the sample we have a record Person, and it has the Age property. Assume that you want to find the person with the highest value for the Age property. We can then use the MaxBy method for that, as you can see here.
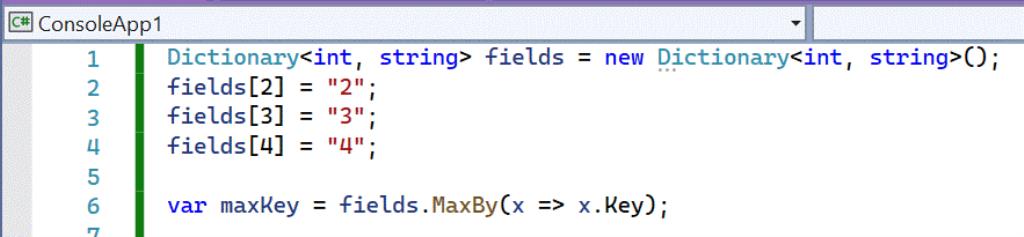
ThrowIfNull
For example, we will in the method need to check if the parameters are null. Instead of that entire ceremony with an if statement, we can now use the more compact ThrowIfNull:
void ThrowIfNullMehod(string arg){
ArgumentNullException.ThrowIfNull(arg);
}
New Async PeriodicTimer
A new timer is also included with .NET 6 called the PeriodicTimer, part of the System.Threading namespace. Using this new timer, we will wait asynchronously for new timer ticks. Here the timer is configured to tick every second, and we wait for that, indeed, asynchronously.
var timer = new PeriodicTimer(TimeSpan.FromSeconds(1));
while(await timer.WaitForNextTickAsync())
{
...
}
Parallel.ForEachAsync()
Using this on an IEnumerable will basically allow us to run multiple concurrent versions of the code in the foreach block This way of processing should be allowing you to go through the list faster as indeed multiple concurrent executions are happening in parallel.
var urls = new[] { "www.pinte.ro", "www.apartamente24.ro" };
var client = new HttpClient();
await Parallel.ForEachAsync(urls, async (url, token) =>
{
HttpResponseMessage responseMessage = await client.GetAsync(url);
});
As part of the .NET 6.0 wave, we are getting a new version of the C# language, version 10. There are quite a few interesting changes that were added to this version of the language, including global usings and file‑scoped namespaces.
IT
.NET 6 new features
03.12.2021
Acasa