In this article I will describe the basic qualities of effective unit tests, and the different kind of testing strategies. Here are the main topics:
1. What is a unit test?
2. Unit tests vs QA Department Testing
3. Qualities of Effective UT
1. WHAT IS A UNIT TEST?
A unit test is a method that instantiates a small portion of our application and verifies its behavior independently from other parts.
A unit test is a piece of a code (usually a method) that invokes another piece of code and checks the correctness of some assumptions afterward.
A unit stands for “unit of work” or a “use case” inside the system.
A unit can span as little as a single method and up to multiple classes and functions to achieve its purpose.
when you test something, you refer to the thing you’re testing as the SUT.
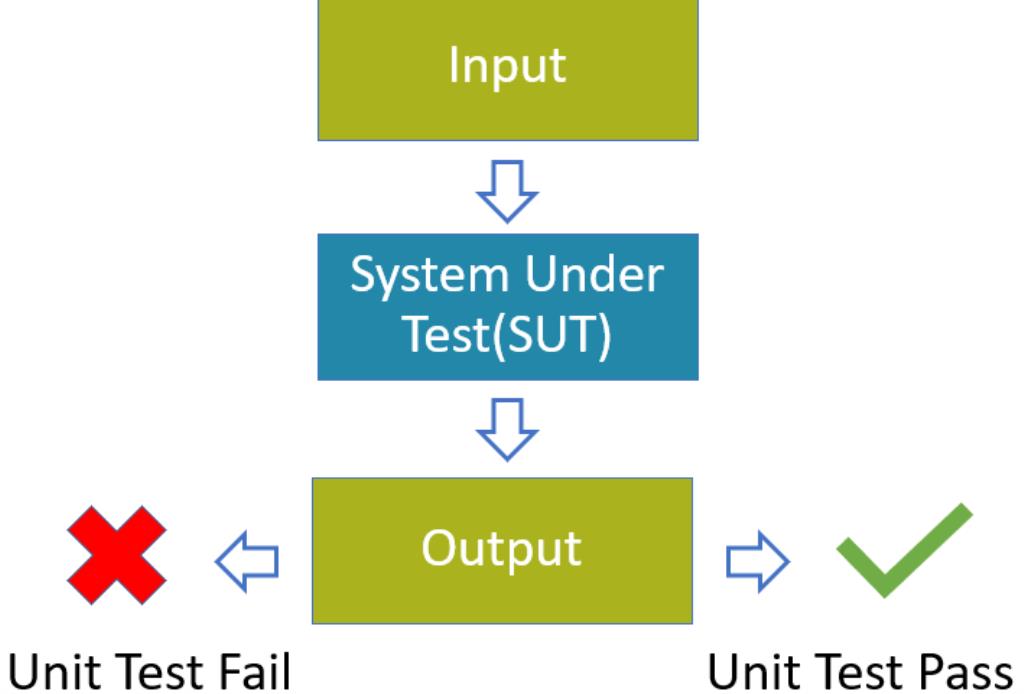
Unit Of Work
A unit of work is the sum of actions that take place between the invocation of a public method in the system and a single noticeable end result by a test of that system.
A noticeable end result can be observed without looking at the internal state of the system and only through its public APIs and behavior. An end result is any of the following:
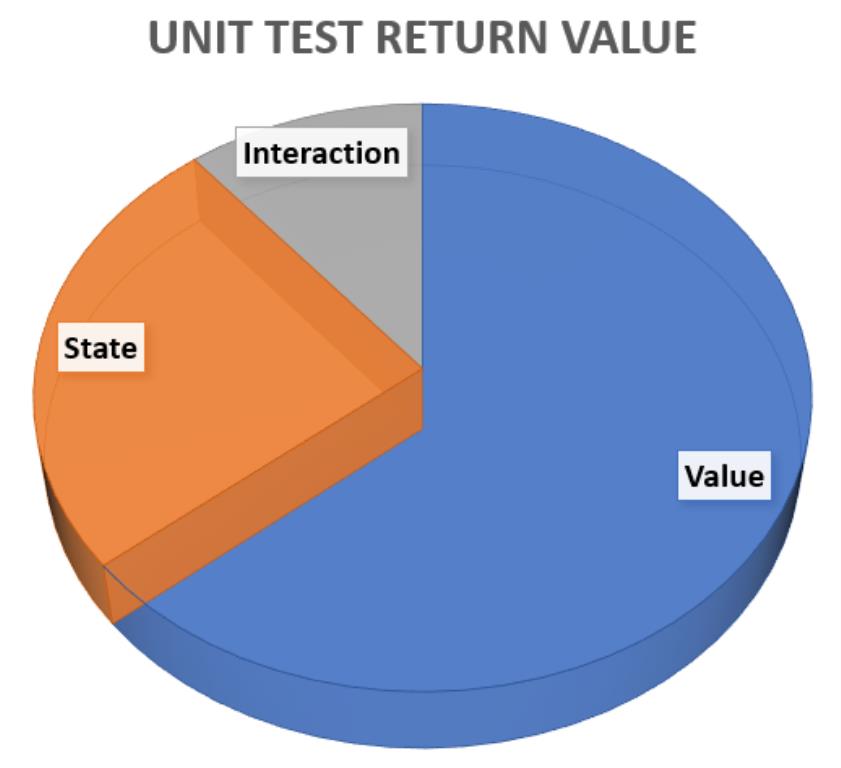
UOW RETURN VALUES
-The invoked public method returns a value
-There’s a state or behavior of the system before and after invocation (Example: the system’s properties change if the system is a state machine.)
-There’s an interaction with a third-party system over which the test has no control, and that third-party system doesn’t return any value. (Example: calling a third-party logging system that was not written by you and you don’t have the source to.)
WHY test code?
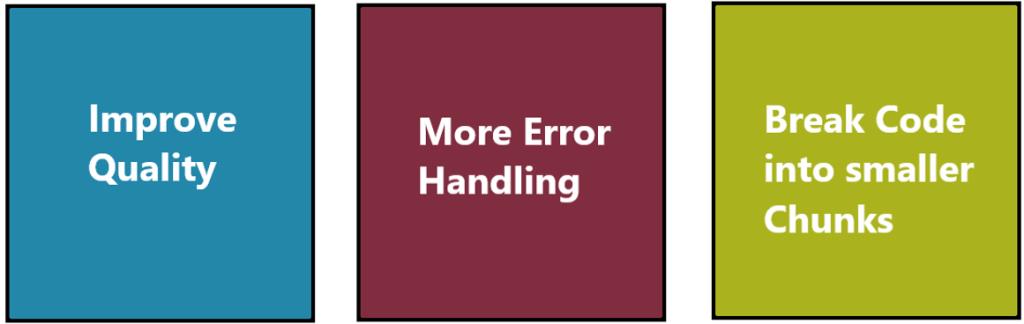
2. UNIT TESTS VS QA DEPARTMENT TESTING
QA Department testing has the following advantages/disadvantages:
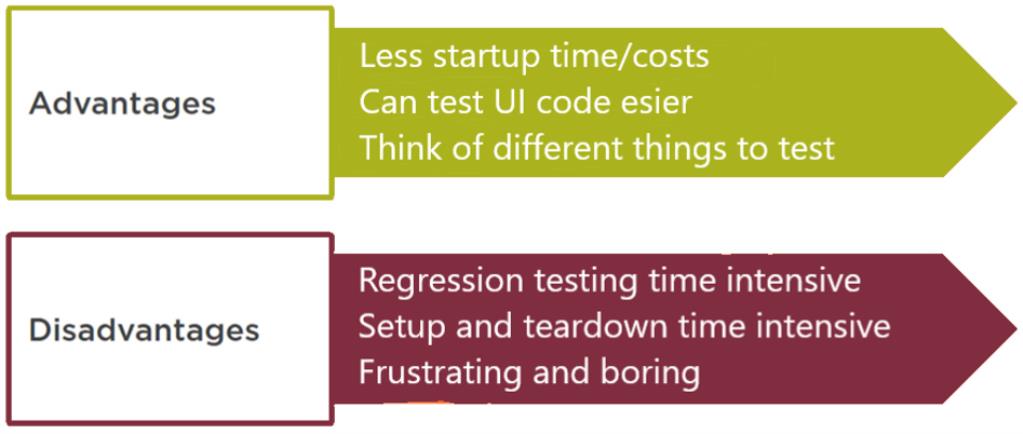
Automated Unit testing has the following advantages/disadvantages:
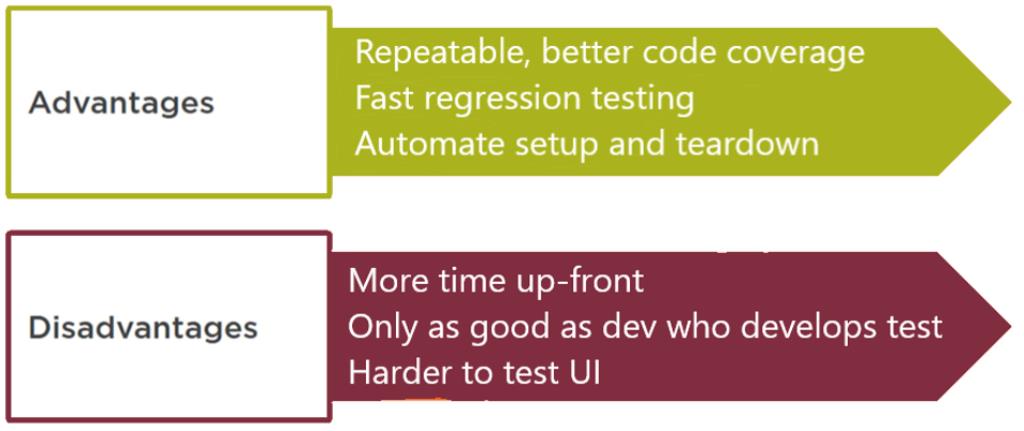
Unit testing doesn’t eliminate QA-related jobs. Instead of doing UI debugging (where every second button click results in an exception of some sort), they’ll be able to focus on finding more logical (applicative) bugs in real-world scenarios.
Unit tests provide the first layer of defense against bugs, and QA work provides the second layer—the user’s acceptance layer.
Allowing the QA process to focus on the larger issues can produce better applications.
3. Qualities of Effective Unit Tests
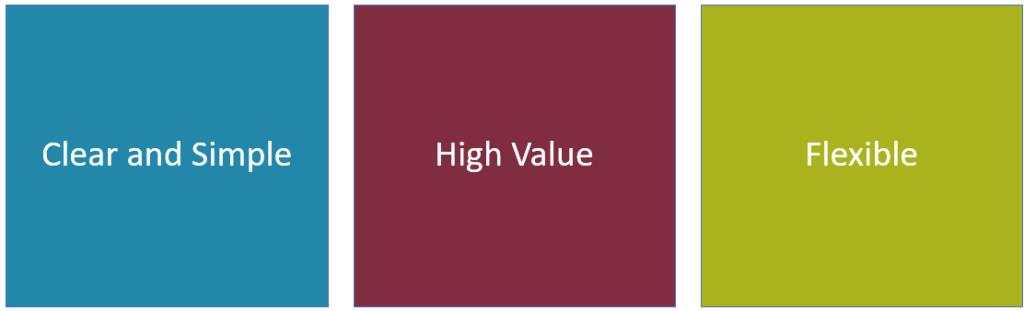
Readable The intent of a unit test should be clear. If a test fails - easy to detect how to address the problem. With a good unit test, we can fix a bug without actually debugging the code!
Independent Tests should not depend on each other. One test should not set up the conditions for the next test. You should be able to run each test independently and run the tests in any order you like.
Fast Tests should be fast. When tests run slow, you won’t want to run them frequently. If you don’t run them frequently, you won’t find problems early enough to fix them easily.
Professional In the long run you'll have as much test code as production (if not more), therefore follow the same standard of good-design for your test code. Well factored methods-classes with intention-revealing names, No duplication, tests with good names, etc.
Truly unit, not integration Unit and integration tests have different purposes. Both the unit test and the system under test should not access the network resources, databases, file system, etc.
AAA Pattern
A typical unit test contains 3 phases:
1. (Arrange) First, it initializes a small piece of an application it wants to test (also known as the system under test, or SUT). Create objects, prepare inputs and configure mocked interfaces.
2. (Act) Perform the actual work of the test, typically by calling a function.
3. (Assert) Verify that the unit under test did what you expected. Did it return the right thing, or throw the correct exception? Ideally this should only require writing a single assert.
UNIT TEST SHOULD HAVE HIGH PRECISION
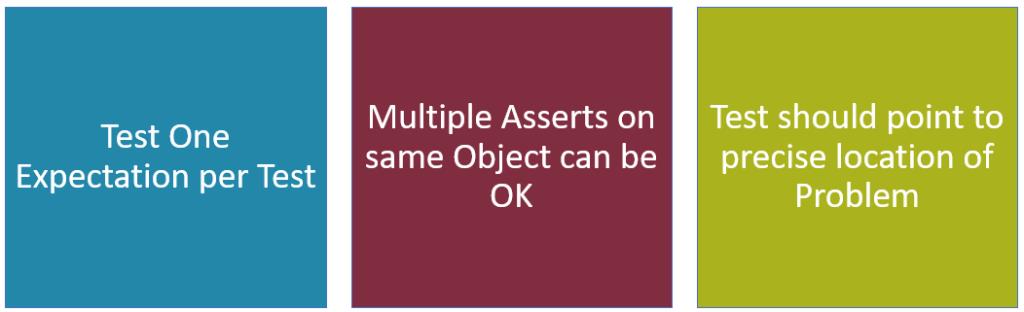
IT
unit testing
16.06.2020
Acasa